Note
Click here to download the full example code
Coding - Decoding simulation of a random messageΒΆ
This example shows a simulation of the transmission of a binary message through a gaussian white noise channel with an LDPC coding and decoding system.
First we create an LDPC code i.e a pair of decoding and coding matrices H and G. H is a regular parity-check matrix with d_v ones per row and d_c ones per column
H, G = make_ldpc(n, d_v, d_c, seed=seed, systematic=True, sparse=True)
n, k = G.shape
print("Number of coded bits:", k)
Out:
Number of coded bits: 11
Now we simulate transmission for different levels of noise and compute the percentage of errors using the bit-error-rate score The coding and decoding can be done in parallel by column stacking.
errors = []
snrs = np.linspace(-2, 10, 20)
v = np.arange(k) % 2 # fixed k bits message
n_trials = 50 # number of transmissions with different noise
V = np.tile(v, (n_trials, 1)).T # stack v in columns
for snr in snrs:
y = encode(G, V, snr, seed=seed)
D = decode(H, y, snr)
error = 0.
for i in range(n_trials):
x = get_message(G, D[:, i])
error += abs(v - x).sum() / (k * n_trials)
errors.append(error)
plt.figure()
plt.plot(snrs, errors, color="indianred")
plt.ylabel("Bit error rate")
plt.xlabel("SNR")
plt.show()
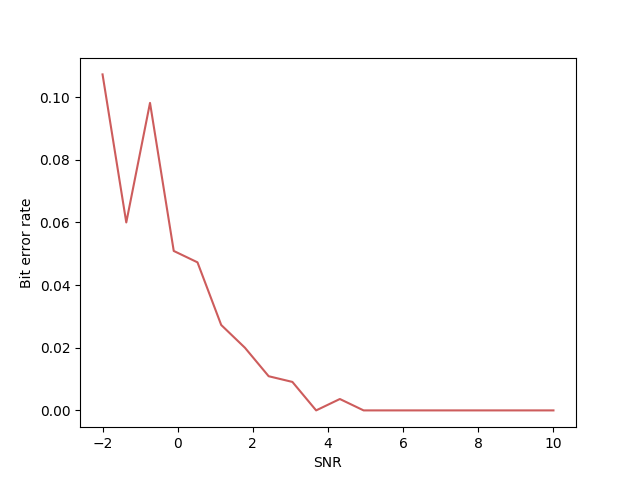
Out:
/Users/hichamjanati/Documents/github/pyldpc/pyldpc/decoder.py:63: UserWarning: Decoding stopped before convergence. You may want
to increase maxiter
to increase maxiter""")
/Users/hichamjanati/Documents/github/pyldpc/examples/plot_coding_decoding_simulation.py:54: UserWarning: Matplotlib is currently using agg, which is a non-GUI backend, so cannot show the figure.
plt.show()
Total running time of the script: ( 0 minutes 2.458 seconds)
Estimated memory usage: 9 MB